Welcome to an extensive exploration of advanced JavaScript operators! This guide is meticulously crafted to delve into Nullish Coalescing, Optional Chaining, and Destructuring Assignment, pivotal tools for enhancing coding efficiency, readability, and error handling in JavaScript.
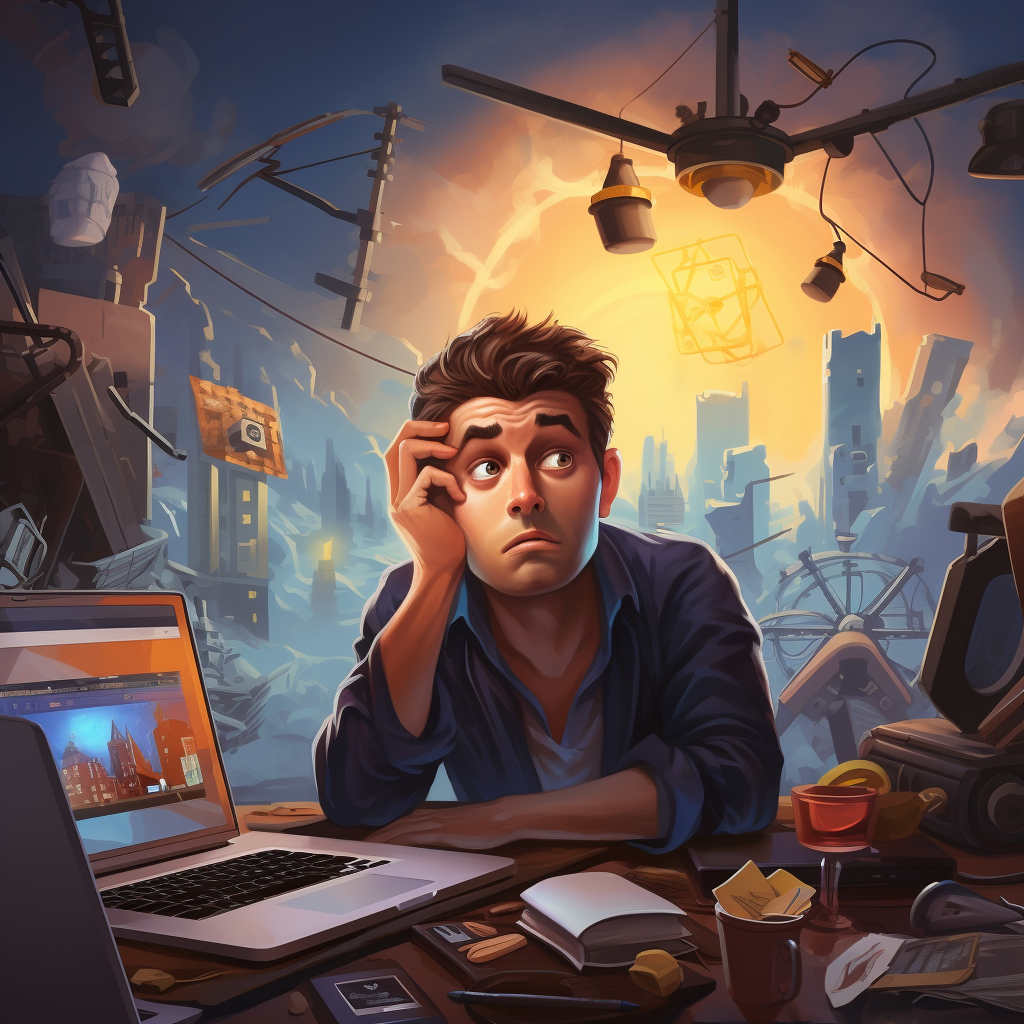
It’s crucial to acknowledge that navigating through these advanced JavaScript operators can be a complex experience, particularly for developers who are newly acquainted with these concepts. The intricacies of Nullish Coalescing, Optional Chaining, and Destructuring Assignment, though highly beneficial in streamlining code and improving error handling, often present a steep learning curve. They require a nuanced understanding of JavaScript’s behavior and syntax. This part of the guide aims to demystify these operators, breaking down their complexity to enhance comprehension, while also highlighting the common challenges and intricacies involved in their application.
Advanced JavaScript Operators: In-Depth Look at Nullish Coalescing Operator (??)
The Nullish Coalescing Operator, a landmark addition in JavaScript ES2020, transforms how developers handle null
or undefined
values. It streamlines conditional logic, making code more concise and clear.
Exploring Nullish Coalescing
Imagine a common scenario in web development where certain user inputs might be missing or undefined:
let userColorChoice = userPreferences.color;
console.log(userColorChoice ?? "Default Color"); // Outputs user's chosen color or "Default Color"
In the example, userColorChoice
defaults to “Default Color” when it’s null
or undefined
, simplifying the traditional if-else logic.
Harnessing the Power of Optional Chaining (?.)
Optional Chaining Operator, another modern addition, is indispensable for safely navigating through nested object structures. It significantly lowers the risk of encountering runtime errors in complex JavaScript applications.
Practical Use of Optional Chaining
Consider a situation where you’re accessing nested user information:
const userProfile = { profile: { name: "Nadav" } };
console.log(userProfile.settings?.notifications?.email); // Outputs undefined instead of an error
This example highlights how Optional Chaining prevents errors if settings
or notifications
are not defined in userProfile
, a common challenge in dynamic data handling.
Mastering Destructuring Assignment for Efficient Data Management
Destructuring Assignment is a sophisticated technique for extracting values from arrays or objects. It’s particularly effective in reducing code verbosity and improving data handling.
Advanced Destructuring Techniques
When working with complex data structures, such as API responses:
const userData = { firstName: 'Nadav', lastName: 'Cohen', email: 'nadav@codama.dev' };
const { firstName, email } = userData;
Here, we efficiently extract firstName
and email
from userData
, showcasing the power of Destructuring in making functions more targeted and comprehensible.
Further Exploration: Advanced Use Cases and Scenarios
These advanced JavaScript operators not only streamline code but also open up new possibilities in handling various scenarios in web development.
Advanced Scenarios for Nullish Coalescing
Beyond simple defaults, Nullish Coalescing can be used in complex conditional chains, making your code more robust against unpredictable data inputs. It’s particularly useful in settings where data integrity is not guaranteed, like user inputs or API responses.
Optional Chaining in Complex Structures
Optional Chaining extends its utility to arrays and function calls. For example, safely accessing an element in an array of objects or conditionally calling a method if it exists. This operator proves its mettle in scenarios involving deeply nested data structures or optional functionality.
Leveraging Destructuring for Enhanced Code Patterns
Destructuring isn’t limited to simple extraction. It can be used in function parameters for more readable and concise function definitions, in swapping variable values without a temporary variable, and in extracting multiple properties simultaneously. This operator is a cornerstone in modern JavaScript patterns, such as React component state and props handling.
Conclusion: The Future of JavaScript with Advanced Operators
In conclusion, Nullish Coalescing, Optional Chaining, and Destructuring Assignment are not just mere additions to JavaScript. They represent a paradigm shift in how JavaScript code is written and understood. These operators, by offering clearer syntax and reducing boilerplate, allow developers to focus more on business logic rather than technical implementation details. As JavaScript continues to evolve, embracing these advanced operators is essential for any developer looking to stay at the forefront of modern web development practices.
Advanced JavaScript Operators – Further Reading and Official Documentation
Here is a list that provides direct links to the MDN (Mozilla Developer Network) documentation for each of the JavaScript operators:
- Nullish Coalescing Operator (??):
MDN Docs on Nullish Coalescing Operator (??) - Logical OR Operator (||):
MDN Docs on Logical OR Operator (||) - Optional Chaining Operator (.?):
MDN Docs on Optional Chaining Operator (.?) - Spread Operator (…): read more here: JavaScript Spread Operator or below in the official MDN Docs:
MDN Docs on Spread Syntax (…)
These links will take you directly to the respective pages on MDN Web Docs, where you can find detailed explanations, syntax, examples, and use cases for each operator.